사람들은 수 많은 지하철역중에 어느 라인(호선)을 이용할까?
11개월의 데이터( 이전포스팅 에서 이야기 한대로 data.go.kr 에 공개된 데이터가 2021.01~2021.11까지 공개되어 있습니다.) 를 가지고 , 본 포스팅에서는 일별 흐름을 중심으로 살펴보겠습니다. 1시간마다 기록되어 있는 승하차 인원중 전체를 살펴보고, 이후 출근 시간(06:00~10:00, 4시간) 과 퇴근시간(17:00~21:00, 4시간)을 중점적으로 살펴보겠습니다.
사용된 툴은 일종의 BI툴이라고 할수 있는 Google Analytics 제품군의 일부인 data studio ( https://datastudio.google.com/ )와 Google Sheet(데이터셋을 업로드) 사용하였습니다.
LINE(호선)별 전체 합계 / 일별 평균 / 시간별 평균 승하차
지난 2021년 01월~2021년 11월까지 1호선~9호선(2~3단계)까지 전체 승하차 인원은 24억 1천만명에 달합니다. 2호선과 1호선(승하차 순위 7위)의 이용객수 차이는 6.14배가 넘습니다. 또한, 2호선이 7호선(승하차 순위 2위) 대비 1.94배가 많아 2호선의 압도적인 수송 규모를 확인할 수 있습니다.
순환 노선인 2호선이 다른 호선에 비해서 압도적으로 많은 이용객수(7억 1천 9백만)가 있다고 볼수 있고, 서울을 북동 <-> 서로 가로지르는 7호선(3억 6천 8백만) 과 북서 <-> 동으로 가로지르는 5호선(3억 3천 6백만)이 뒤를 이어 이용객수가 많습니다.
일별 평균을 보면 하루평균 약 721만명이 지하철을 이용하고 있고, 2호선은 일평균 2백만명이상(시간당 11만명이상), 7호선은 110만명(시간당 5만 8천명이상), 5호선은 100만명(시간당 5만 3천명이상)이 일일 평균 이용함을 알수 있습니다. 다른 노선들은 일평균 82만명을 하회하고 있습니다. 2호선의 혼잡도가 압도적으로 높다는 것을 수치로 확인 할 수 있습니다.
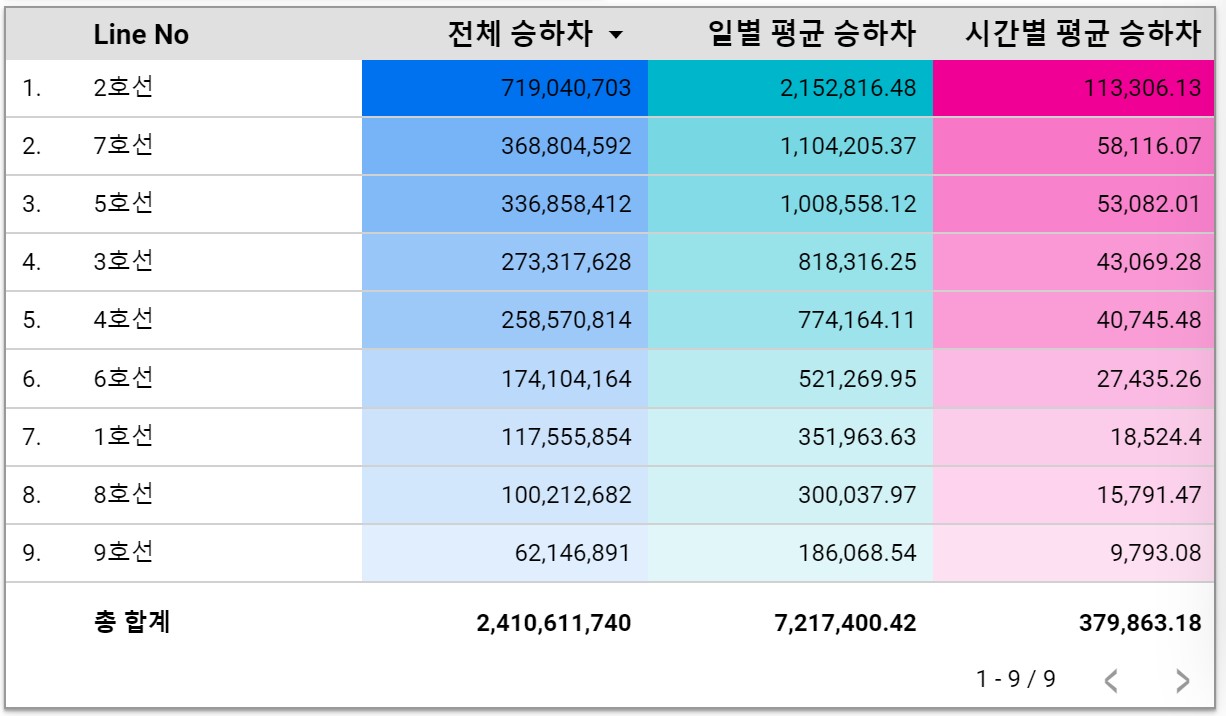
: 11개월동안 전체 승하차 인원표
승차(승차/환승승차) 와 하차(하차/환승하차)를 각각 분리하여 보면 다음과 같습니다. 승차 인원은 전체 12억 5백9십만정도이고, 하차 인원은 12억 4백6십만 정도입니다. 승차를 했으면 하차를 해야 하는데 이 수치가 130만정도가 차이가 나는데, 이것은 9호선 1~2단계 구간(민자구간)과 중앙선, 분당선, 신분당선등 다른 노선으로 하차한 승객들 때문일 것으로 보입니다. 이 비중은 1%안쪽으로 비중이 크지 않습니다.
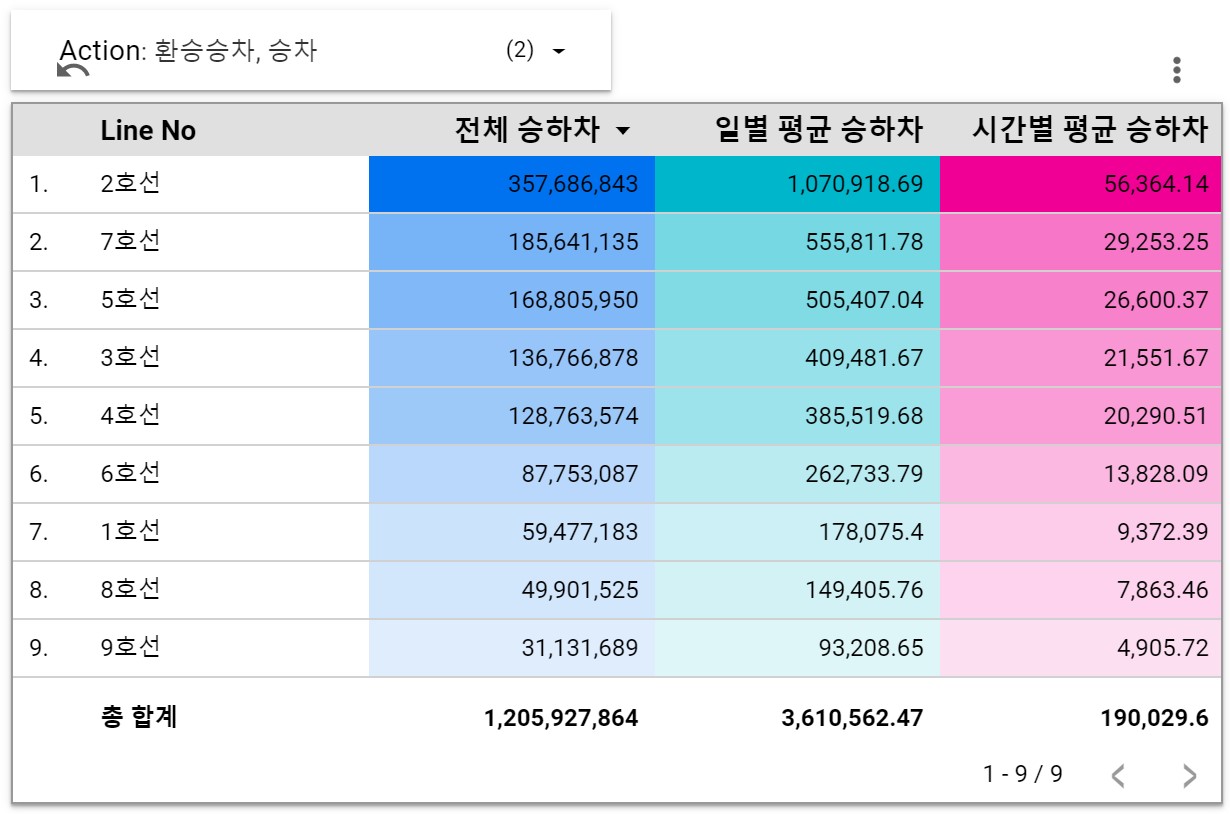
: 11개월동안 전체 승차인원표
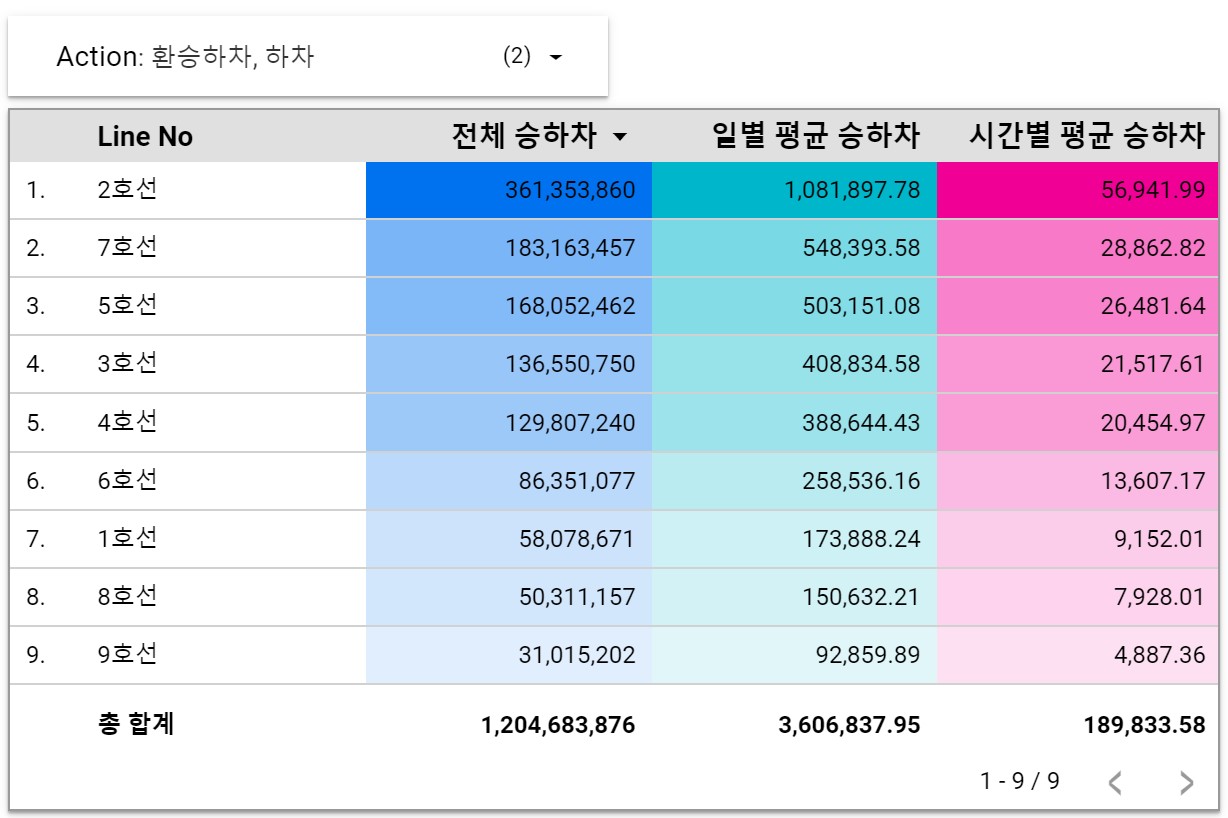
: 11개월동안 전체 하차 인원표
출퇴근 시간대 Trend
[출근 시간대-승하차 Trend]
11개월동안의 일별 승하차 trend를 출근시간대로 한정하여 살펴보면 아래와 같습니다.
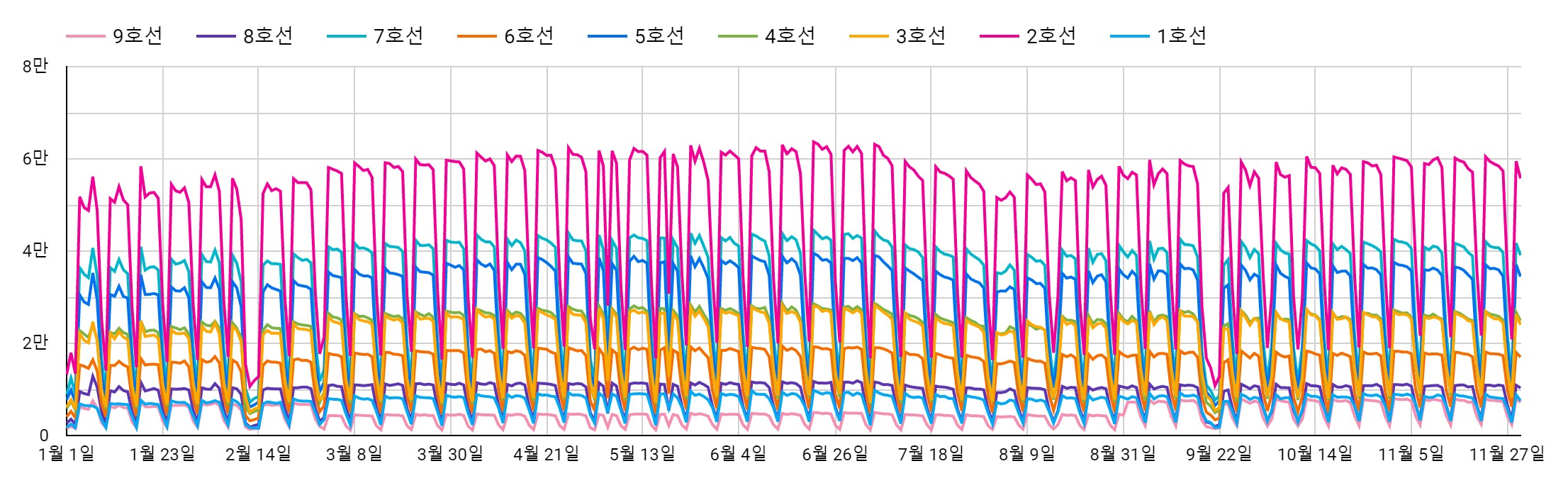
: 일자별(date)로 본 06~07시 승하차 노선별 현황
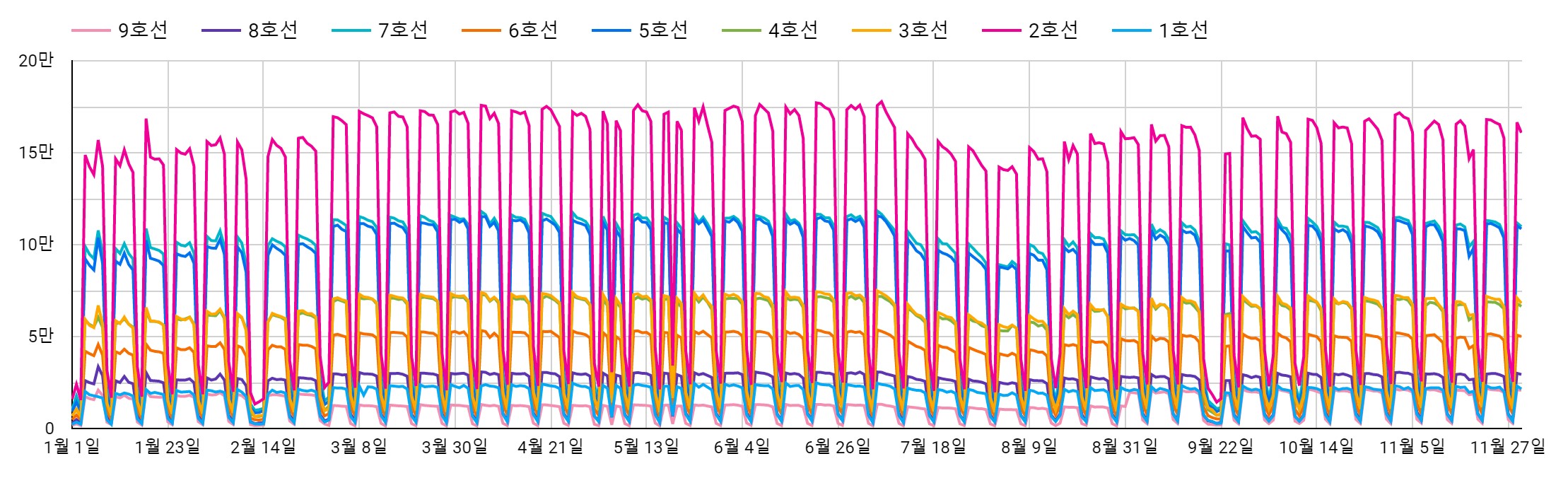
: 일자별(date)로 본 07~08시 승하차 노선별 현황
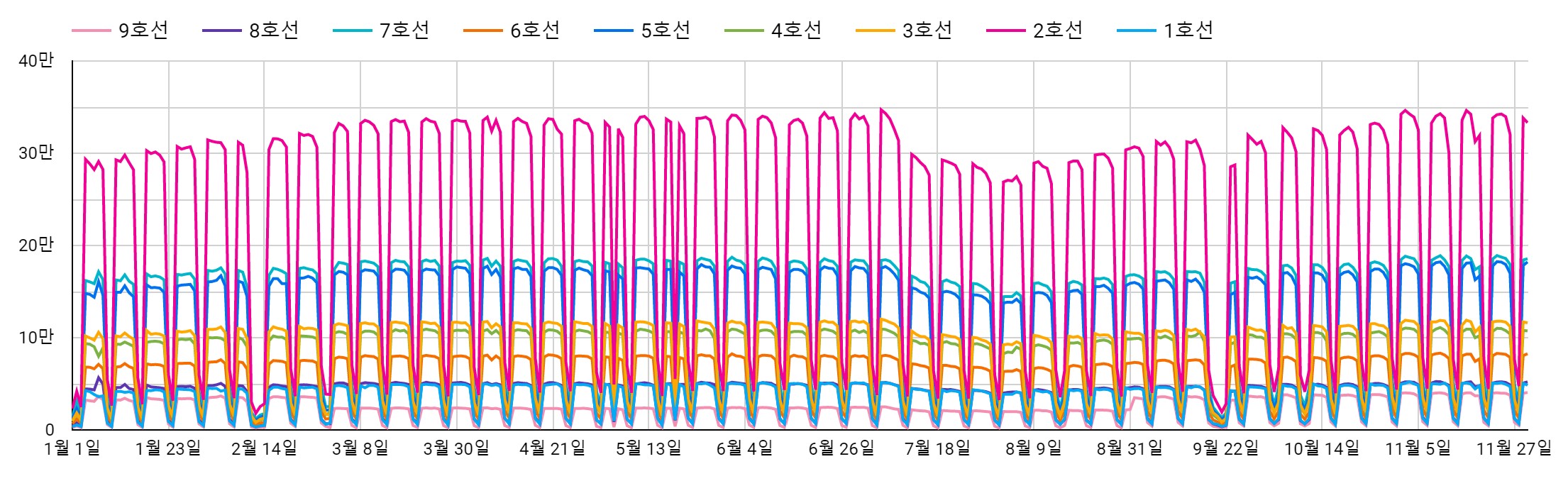
: 일자별(date)로 본 08~09시 승하차 노선별 현황
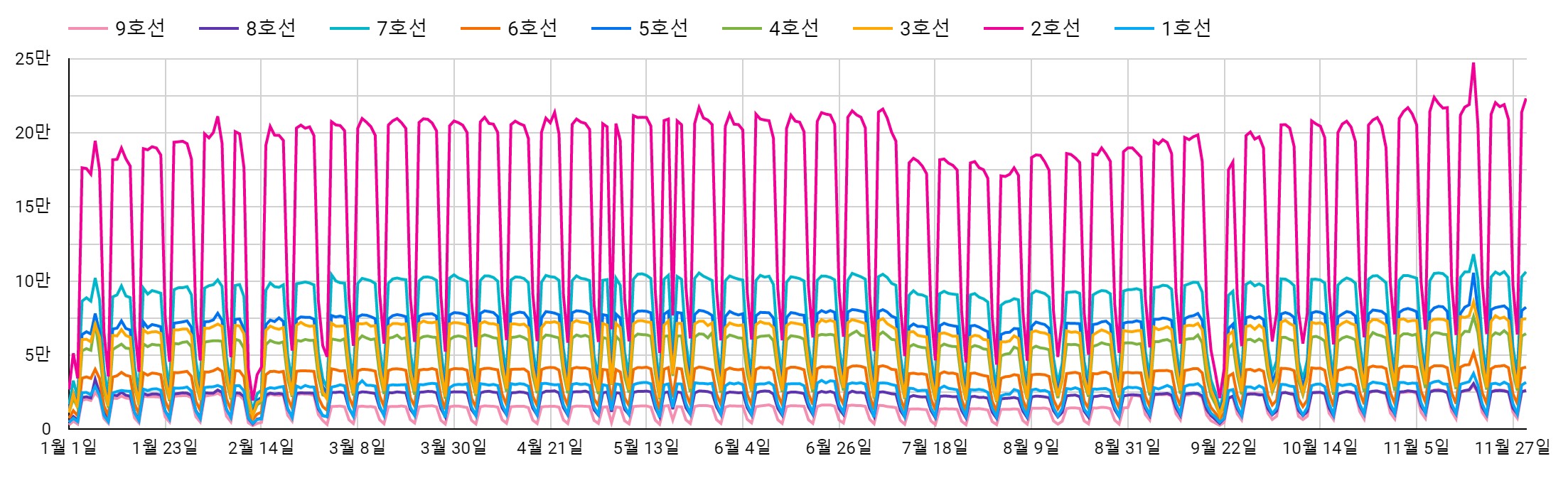
: 일자별(date)로 본 09~10시 승하차 노선별 현황
위 그래프에서 약 4시간(06:00~10:00) 출근 시간동안 비슷한 패턴을 보이는데 2->7->5->3->4->6->8->1->9 호선 같은 순서로 사람들이 승하차를 많이 함을 알수 있습니다. 앞선 포스팅에서 이야기 하였듯이 9호선은 2~3단계 노선의 승하차 인원만 존재하여 가장 순위가 낮지만 실제로는 상위순위일 것으로 예상됩니다.
위 그래프에서 출근시간대에 7월~8월 중순까지 그래프가 움푹 파인 구간이 있는데 이 구간은 여름휴가 기간동안 승하차 인원이 감소하는 것으로 생각할 수 있습니다.
[퇴근 시간대-승하차 Trend]
11개월동안의 일별 승하차 trend를 퇴근시간대로 한정하여 살펴보면 아래와 같습니다.
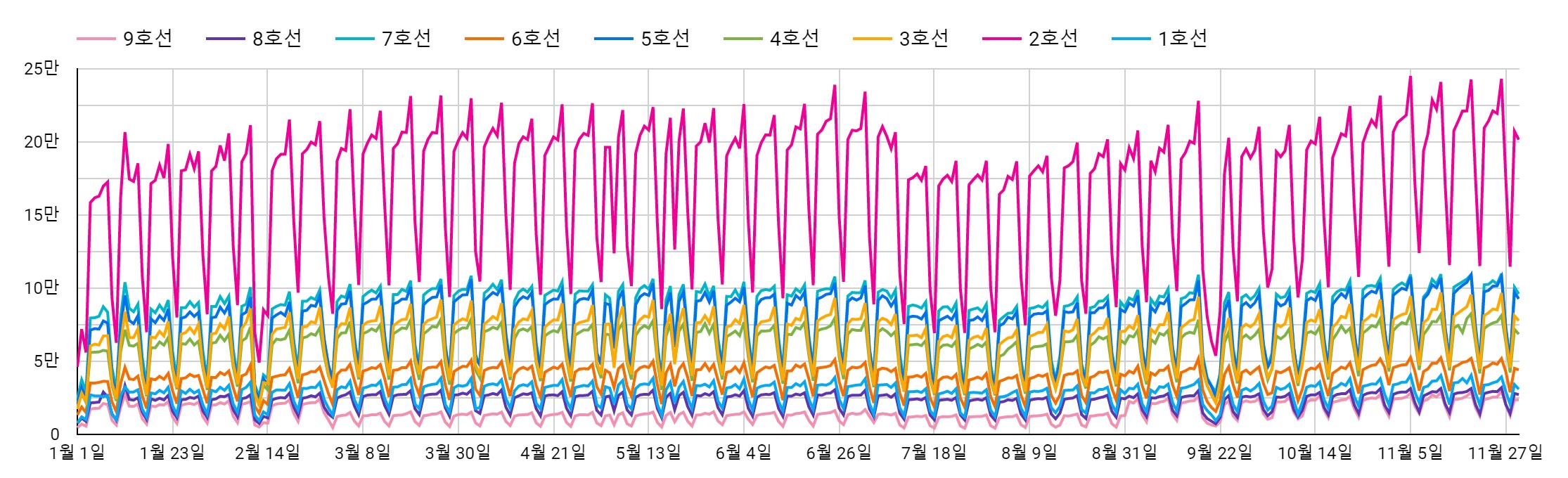
: 일자별(date)로 본 17~18시 승하차 노선별 현황
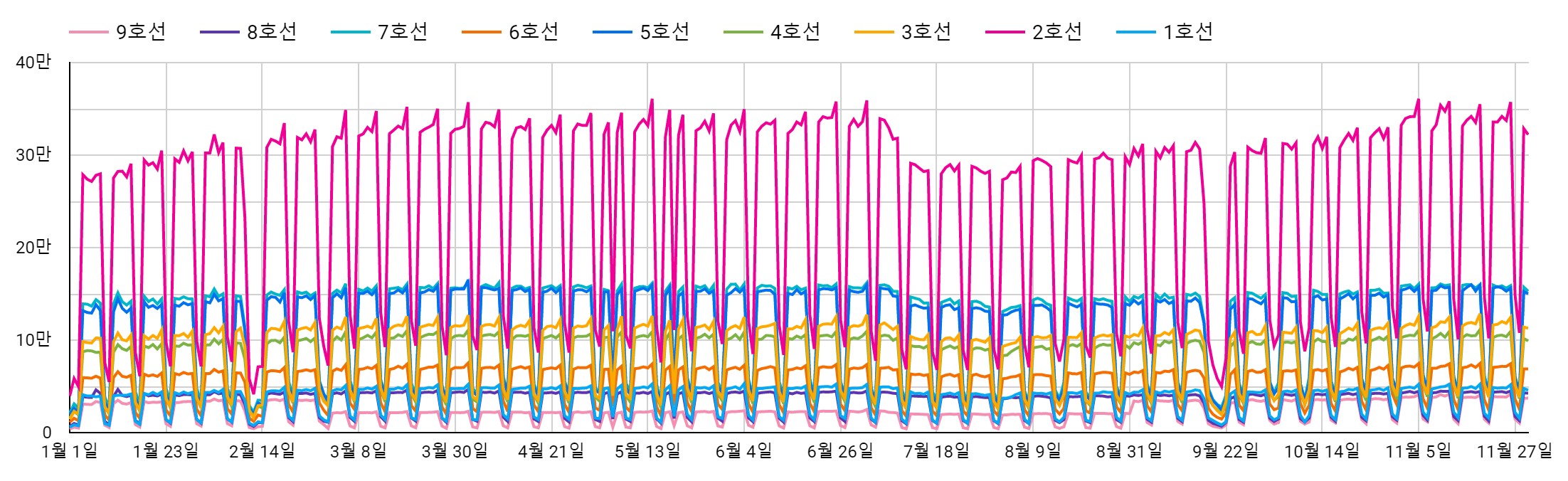
: 일자별(date)로 본 18~19시 승하차 노선별 현황
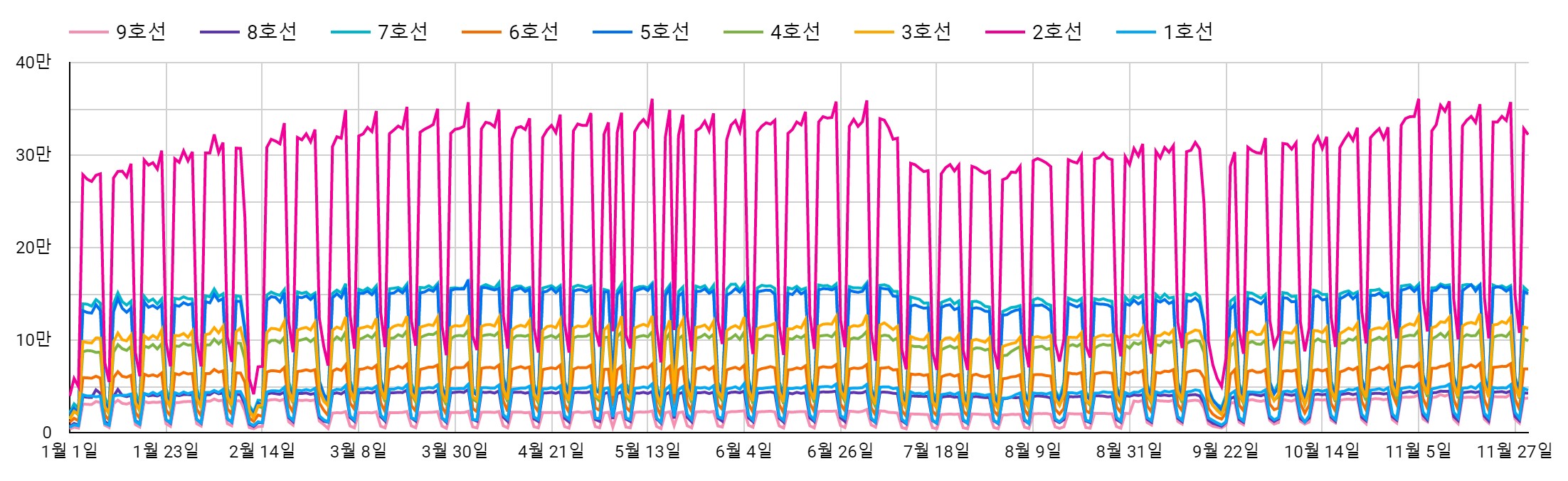
: 일자별(date)로 본 19~20시 승하차 노선별 현황
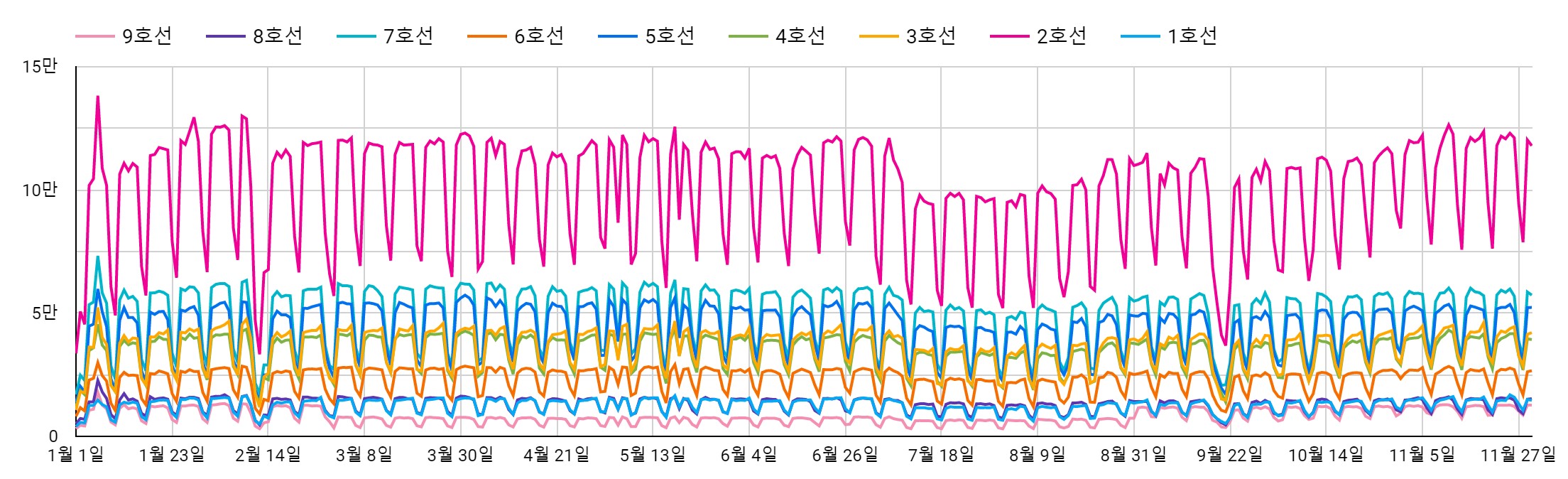
: 일자별(date)로 본 20~21시 승하차 노선별 현황
퇴근시간은 출근시간대와 달리 1호선이 8호선 보다 승하차 인원이 많아 순위가 바뀐 2->7->5->3->4->6->1->8->9 호선 같은 순서로 사람들이 승하차를 많이 합니다. 출근시간대와 마찬가지로 퇴근시간대에 7월~8월 중순까지 그래프가 움푹 파인 구간이 있는데 이 구간 역시 여름휴가 기간동안 오전 시간대와 마찬가지로 승하차 인원이 감소하는 것으로 생각할 수 있습니다.
출퇴근 시간대 합계
[출퇴근 시간대-승하차 합계]
아래표는 11개월동안 출근 시간 구간의 승하차 인원의 전체 합계입니다.
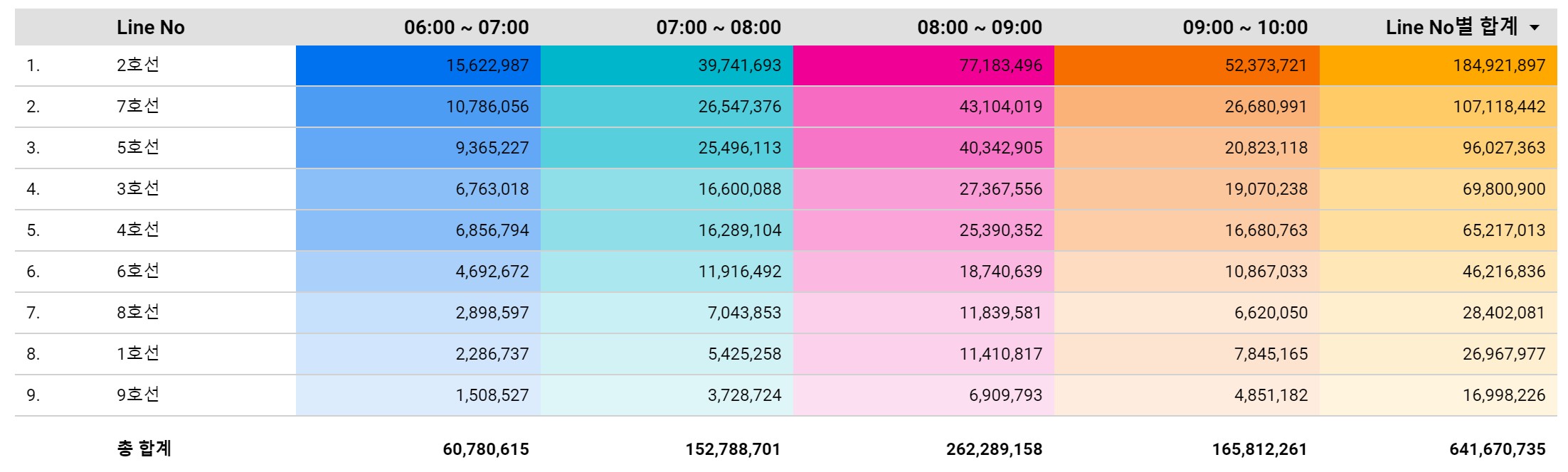
: 오전 출근시간동안 승하차 인원표
약 4시간에 달하는 출근 시간동안 전체 승하차인원(24억 1천만)의 약 27%(6억 4천 1백만)가 집중되고 있습니다. 08:00~09:00시간대에 승하차인원(2억 6천 2백만, 11%)이 가장 많음을 알수 있습니다. 09:00~10:00까지도 이러한 경향은 계속된다고 볼수 있습니다.
아래표는 11개월동안 퇴근 시간 구간의 승하차 인원의 전체 합계입니다.
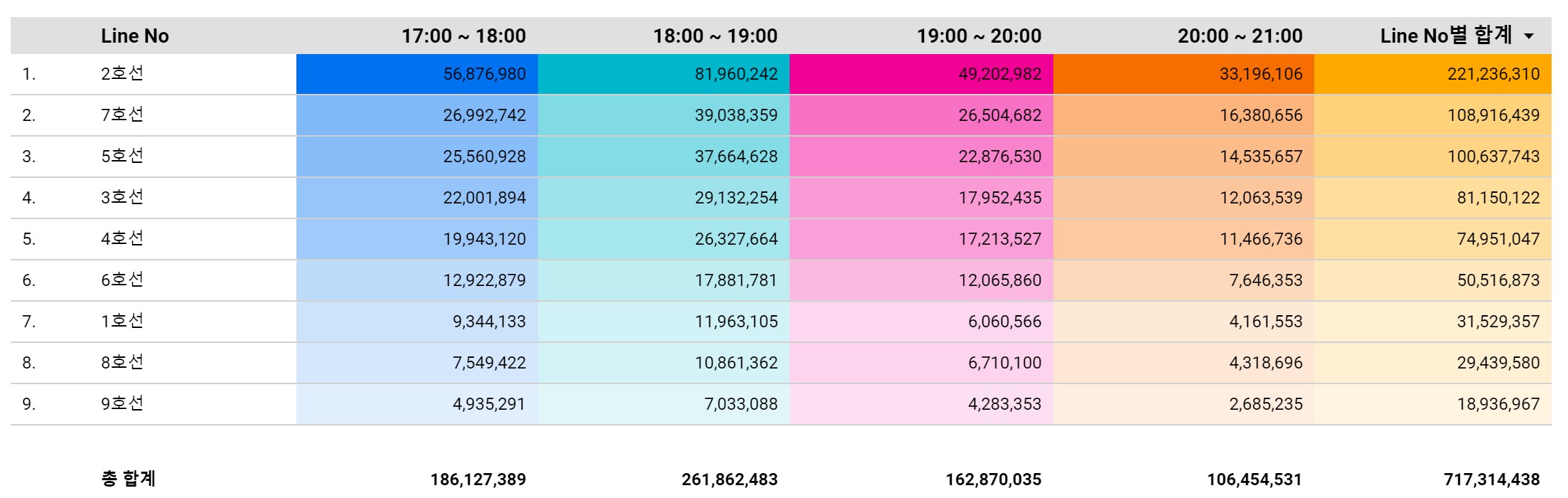
: 오후 퇴근시간동안 승하차 인원표
약 4시간에 달하는 퇴근 시간동안 전체 승하차인원(24억 1천만)의 약 30%(7억 1천 7백만)가 집중되고 있습니다. 18:00~19:00시간대에 승하차 인원(2억 6천 1백만, 11%)이 집중되고 있습니다. 19:00~20:00 보다 17:00~18:00의 수치가 더 높아 이때 퇴근이 더 많을 것이라고 예상할 수 있습니다.
위 표들은 승하차인원의 합계이기 때문에, 이를 상세화 하면 다음과 같이 승하차를 구분지어 생각할 수 있습니다.
- 아침 출근 시간대
- 승차 : 거주지 근처 역에서 승차하는 경우 , 버스에서 내린 후 지하철에 승차하는 경우
- 하차 : 주로 일을 하게 되는 사무실 주변역에서 하차하는 경우
- 저녁 퇴근 시간대
- 승차 : 주로 일을 하게 되는 사무실 주변역에서 하차하는 경우
- 하차 : 거주지 근처역에서 하차하는 경우 , 버스를 타기 위해 지하철에서 하차 하는 경우
위와 같은 관점으로 승차, 하차를 분리하여 확인해 보겠습니다.
[출퇴근 시간대-승차 합계]
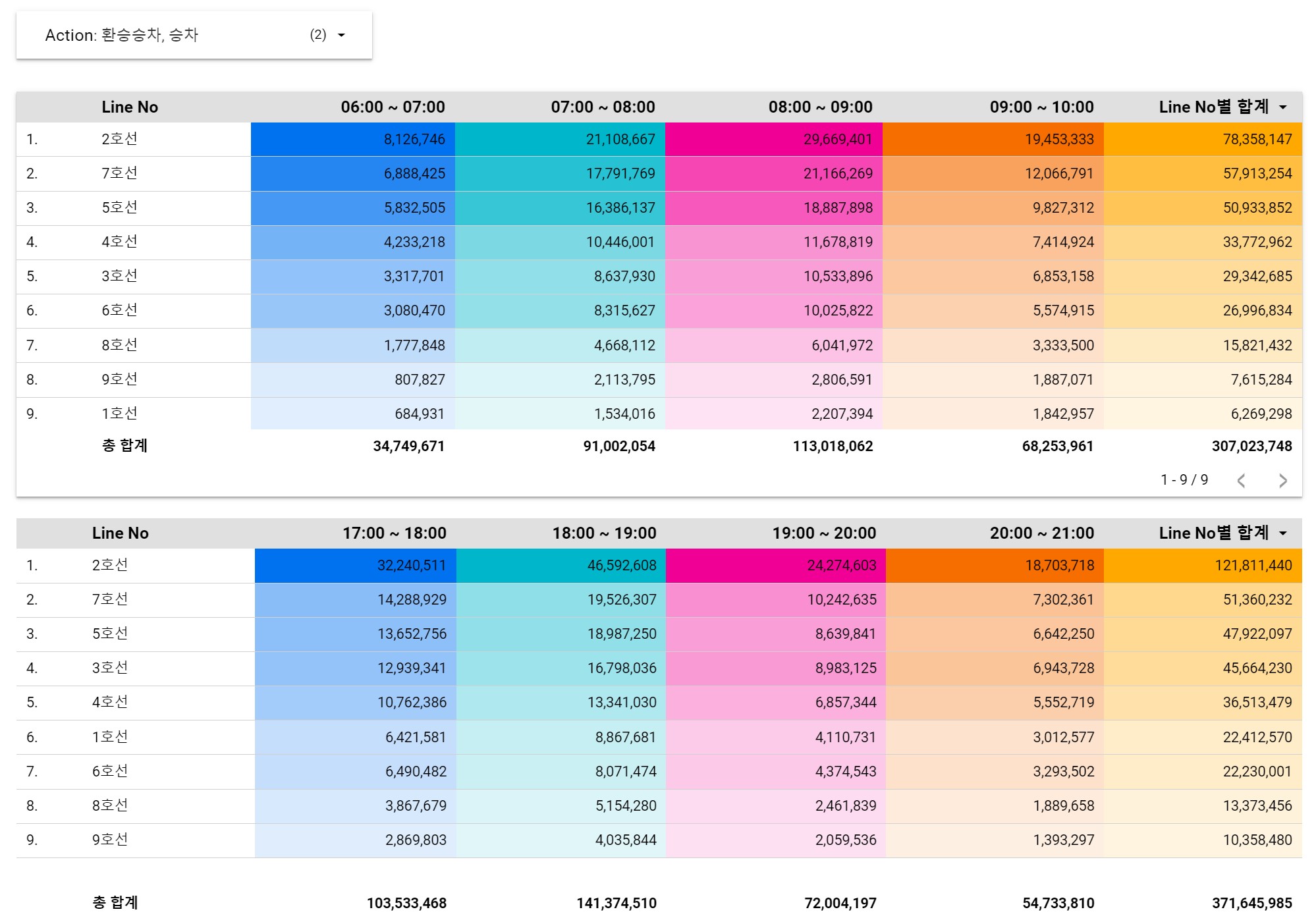
출근 시간대 승차만을 살펴보면 전체 승하차와 비교했을때 3호선(합계 2천 9백만) 과 4호선(합계 3천 3백만) 의 순위가 바뀌고, 1호선(합계 6백 2십만)과 9호선(합계 7백 6십만)의 순위가 바뀌게 됩니다. 4호선이 3호선보다 출근을 위해 거주지 근처 지하철을 이용하는 사람이 많다고 볼수 있습니다. 9호선은 2~3단계 구간임에도 승차 인원이 1호선보다 많다는 것은 김포공항 방향에서 출발하여 출근하는 인원이 많다는 것을 알수 있습니다.
퇴근 시간대 승차만을 살펴보면 1호선의 순위가 출근시간대 9위(합계 6백 2십만)에서 퇴근시간대 6위(합계 2천 2백 4십만)가 되는 것은 1호선이 거주보다는 직장에서 퇴근을 위해 승차하는 사람이 상대적으로 많다는 것으로 추정할 수 있습니다.
출근 시간대, 퇴근시간대 전체 승차 인원은 각각 3억 7백만, 3억 7천 1백만으로 전체 승하차 인원(24억 1천만)의 12.74%, 15.42%를 점유합니다.
[출퇴근 시간대-하차 합계]
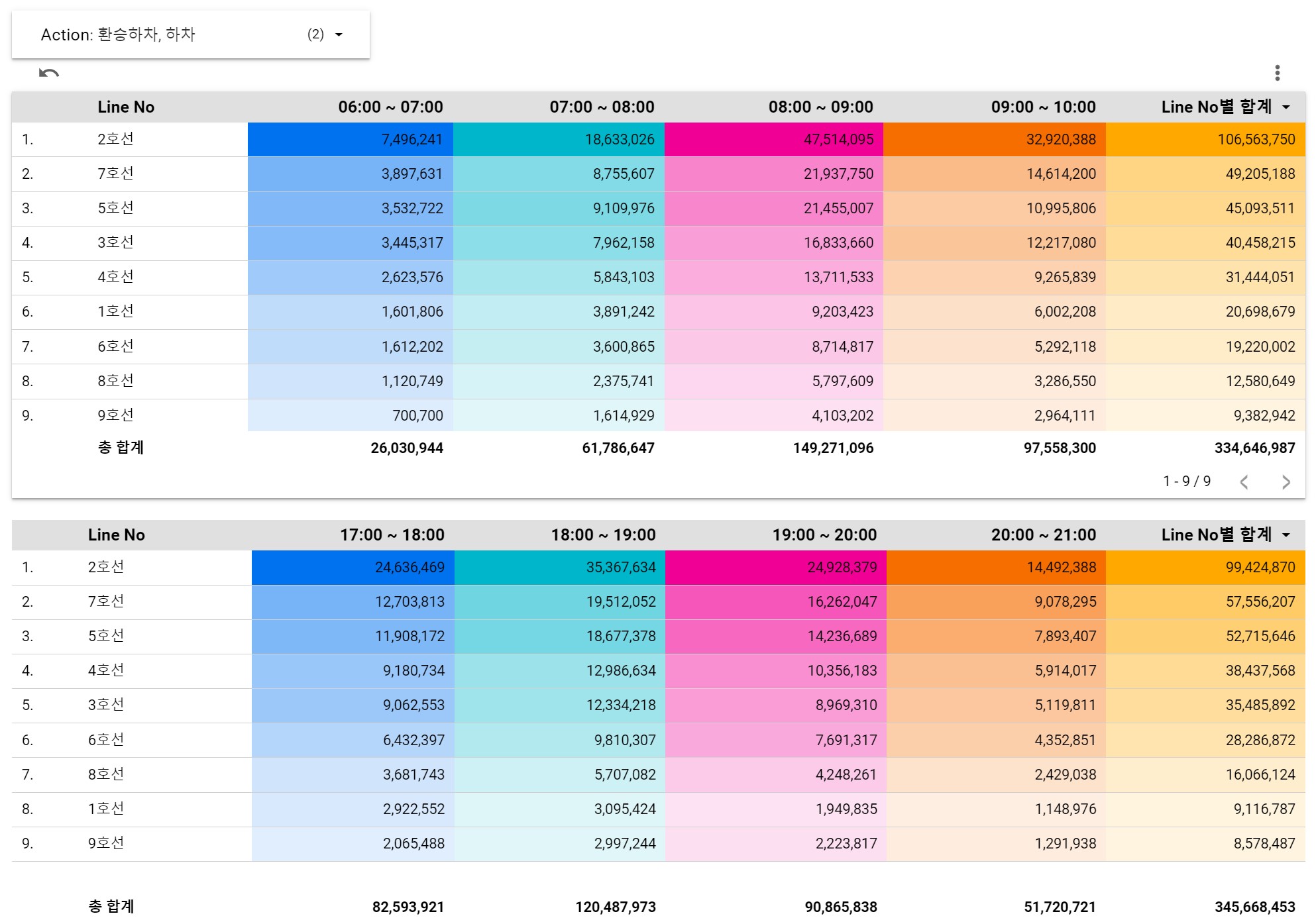
앞서 출퇴근 시간대 승차인원을 통해 언급했던 “1호선은 거주보다는 직장에서 퇴근을 위해 승차를 많이 한다.”는 내용은 출퇴근 시간대 하차에서도 확인 할 수 있습니다. 1호선의 출근 시간대 하차 순위가 6위(합계 2천 6십만) 였다가, 퇴근시간대 하차 순위는 8위(합계 9백 1십만)로 떨어지는 것을 볼수 있습니다. 아침시간대 출근을 위해 하차 한 인원들이 상대적으로 많다고 볼수 있습니다.
출근 시간대, 퇴근시간대 전체 하차 인원은 각각 3억 3천 4백만, 3억 4천 5백만으로 전체 승하차 인원(24억 1천만)의 13.88%, 14.34%를 점유합니다.
전체적으로 2, 7, 5 호선은 출근시간대의 승차 순위(2->7->5), 퇴근시간대의 하차 순위(2->7->5)가 전체 승하차 순위(2->7->5)와 동일하여 거주지가 집중되고, 직장도 많이 분포한다고 추정할 수 있습니다. 다른 라인에서는 특히 1호선이 거주 보다는 직장이 분포된 라인이라고 추정할 수 있습니다.
지하철 승하차 순위와 이용 분포를 통해서 주택가격이 이러한 지하철 이용객 분포와 연결이 되지 않을까 가정(실제 영향을 크게 미치겠지만)할 수 있는데 이는 향후 추가적으로 살펴보도록 하겠습니다.
- 다음 포스팅에 이어집니다. -